In the world of container orchestration, mastering the Kubernetes code base is essential for developers and DevOps engineers alike. To get started with Kubernetes, check out our guide on Kubernetes Basics for a thorough introduction.
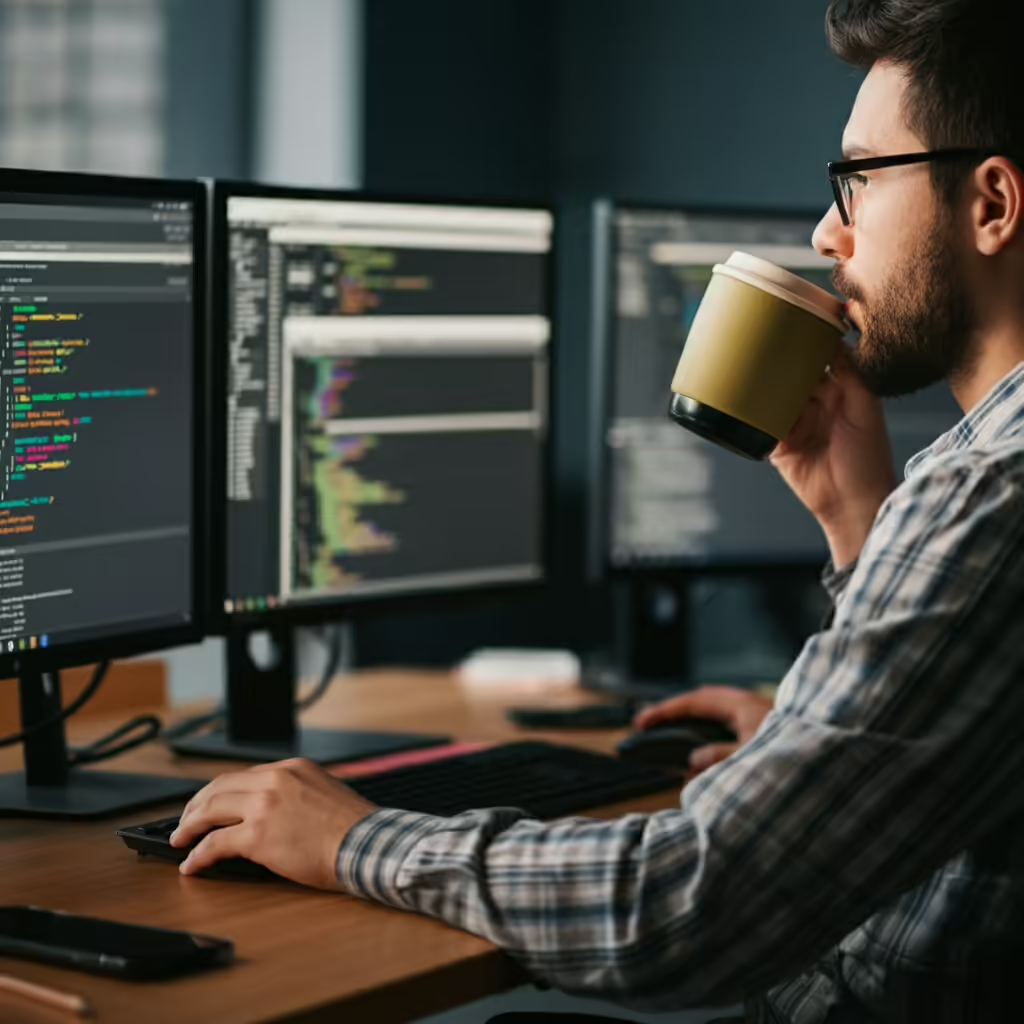
- Introduction
- Why Learn the Kubernetes Code Base
- Understanding the Kubernetes Repositories
- Running a Basic kubectl Command
- Locating Command Implementations in the Source Code
- Navigating the Code with Builders and Visitors
- Compiling and Running Kubernetes
- Essential Tools and Techniques for Learning the Code Base
- Conclusion
Introduction
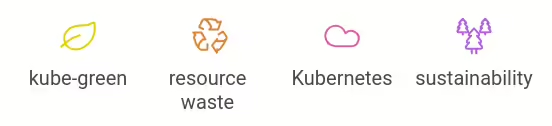
Mastering the Kubernetes code base is a significant milestone for any developer aiming to contribute to one of the most impactful open-source projects today. This guide breaks down the complexities and provides a step-by-step approach to understanding and navigating the Kubernetes source code effectively.
Why Learn the Kubernetes Code Base
Kubernetes continues to experience explosive growth, and developers proficient in its code base are in high demand. Learning the Kubernetes code base empowers you to contribute to the project, optimize deployments, and enhance your problem-solving skills in cloud-native environments.Understanding the Kubernetes Repositories
The Kubernetes code base is organized across several repositories:- kubernetes/kubernetes: Contains the main() function of the kubectl command. It’s the core repository used to build kubectl. https://github.com/kubernetes/cli-runtime
- kubernetes/kubectl: Houses the implementation of each kubectl subcommand, such as create.
- kubernetes/cli-runtime: Provides helper functions used by kubectl, like resource.Helper.
- Understand the Directory Structure
The Kubernetes project follows a standard Go project structure with two key directories:
cmd
: Contains the main application packagespkg
: Contains reusable libraries and utilities
- Explore Key Components
Start exploring by looking at some of the key components:
kube-apiserver
: The core API server of Kuberneteskube-controller-manager
: Manages controller processeskube-scheduler
: Schedules pods onto nodes
Each component has its own directory under
cmd
with a main package (e.g.,apiserver.go
,controller-manager.go
). - Follow Function Calls
To understand how components interact:
- Start in the main package of a component
- Follow function calls to other packages
- Examine interfaces and custom types used by each component
For example, in
kube-apiserver
, follow frommain()
toNewAPIServerCommand()
and then into theapp
package. - Each command corresponds to a folder, e.g., the create command is in create/create.go.
- Kubernetes uses the Cobra command framework, which structures commands and their descriptions cohesively.
- Builders: Functions like Unstructured, Schema, and ContinueOnError modify and return a Builder object.
- Visitors: The Do() function of the Builder returns a Result object containing a Visitor, which traverses the list of resources.
- Follow the on-screen instructions to add
etcd
to yourPATH
. - You can add fmt.Printf() statements in the code to verify execution paths.
- What It Does: Provides IDE-like features in your browser when viewing code on GitHub.
- Benefits: Hover over functions to see definitions, references, and documentation without leaving the page.
- Get It Here: Chrome Web Store – Sourcegraph
- Purpose: Validate the flow of execution and inspect variables.
- Tip: Use fmt.Printf(“Variable info: %#v\n”, variable) for detailed output.
- How: Insert panic(“Debugging stack trace”) at critical points.
- Why: Generates a stack trace, helping you understand the call hierarchy.
- Use Case: Identify when and why a particular piece of code was changed.
- How: Click on the Blame button in a GitHub file view to see commit history line by line.
- Kubernetes GitHub Repository (Kubernetes)
- Cobra Command Framework (Cobra)
- Chrome Sourcegraph Extension (Chrome Sourcegraph)
git clone https://github.com/kubernetes/kubernetes.git
cd kubernetes
Running a Basic kubectl Command
We’ll explore the flow of the kubectl create -f
command, which creates a resource from a file. Here’s an example YAML file (nginx_pod.yaml
) that defines a single-replica pod running an Nginx container:
apiVersion: v1
kind: ReplicationController
metadata:
name: nginx
spec:
replicas: 1
selector:
app: nginx
template:
metadata:
name: nginx
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx
ports:
- containerPort: 80
Run the command:
kubectl create -f ~/nginx_kube_example/nginx_pod.yaml
Locating Command Implementations in the Source Code
The entry point for kubectl commands is in the pkg/cmd directory:Navigating the Code with Builders and Visitors
The RunCreate function employs method chaining with builders to process command-line arguments:Compiling and Running Kubernetes
To experiment with the code:Compile Only kubectl
to Save Time:
make WHAT='cmd/kubectl'
Install etcd
:
KUBERNETES_PROVIDER=local hack/install-etcd.sh
Start the Kubernetes Cluster Locally:
Install etcd
:
KUBERNETES_PROVIDER=local hack/local-up-cluster.sh
Run the Modified kubectl
Command:
cluster/kubectl.sh create -f ~/nginx_kube_example/nginx_pod.yaml
Essential Tools and Techniques for Learning the Code Base
Chrome Sourcegraph Extension
Effective Use of Print Statements
Using panic() for Stack Traces
Leveraging GitHub’s Blame Feature
Conclusion
Mastering the Kubernetes code base is a rewarding endeavor that enhances your development skills and opens up opportunities in the cloud-native landscape. By systematically exploring the repositories, understanding command implementations, and utilizing essential tools, you can demystify the complexities of Kubernetes.Every journey begins with a single step. Start exploring the Kubernetes code base today!
Kubernetes Links: External Resources: