As AI models grow in size and complexity, tools like vLLM and Ollama have emerged to address different aspects of serving and interacting with large language models (LLMs). While vLLM focuses on high-performance inference for scalable AI deployments, Ollama simplifies local inference for developers and researchers. This blog takes a deep dive into their architectures, use cases, and performance, complete with code snippets and benchmarks.
What is vLLM?
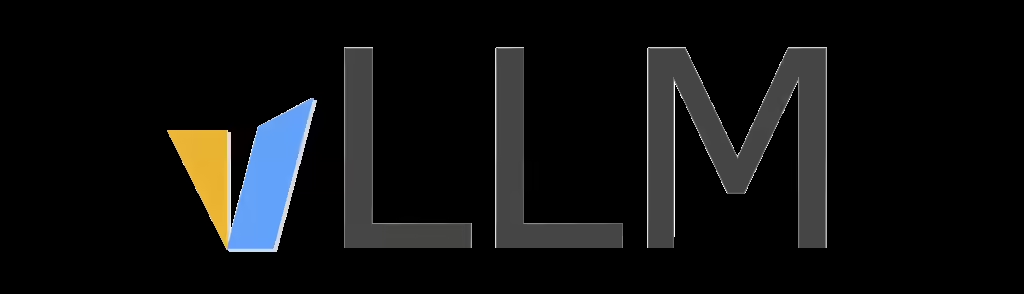
vLLM is an optimized serving framework designed to deliver low-latency and high-throughput inference for LLMs. It integrates seamlessly with distributed systems, making it ideal for production-grade AI applications.
Primary Goal: Serve high-performance AI workloads with efficiency and scalability.
What is Ollama?
Ollama is a developer-friendly tool designed for running LLMs locally. By prioritizing simplicity and offline usage, it empowers developers to prototype and test models without the overhead of cloud infrastructure.
Primary Goal: Enable local AI inference with minimal setup and resource consumption.
Key Features
Feature | vLLM | Ollama |
---|---|---|
Deployment Mode | API-based, distributed | Local inference |
Performance | High throughput, low latency | Optimized for small-scale, offline |
Hardware Utilization | Multi-GPU, CPU, and memory optimized | Single-device focus |
Ease of Use | Requires server setup | Ready-to-use CLI |
Target Audience | Production teams | Developers and researchers |
Highlight
- Local Inference: Ollama excels in environments where simplicity and privacy are paramount.
- Scalability: vLLM shines in large-scale AI deployments requiring parallel requests and distributed processing.
Code Snippets
1. Loading a Pre-trained Model with Ollama
import subprocess
def run_ollama(model_name, prompt):
"""
Run a prompt against a local Ollama model.
"""
result = subprocess.run(
["ollama", "run", model_name],
input=prompt.encode(),
stdout=subprocess.PIPE,
text=True
)
return result.stdout
# Example usage
response = run_ollama("gpt-neo", "What are the benefits of local AI inference?")
print(response)
2. Serving a Model with vLLM
import requests
def query_vllm(api_url, model_name, prompt):
"""
Send a prompt to a vLLM API endpoint.
"""
payload = {
"model": model_name,
"prompt": prompt,
"max_tokens": 100
}
response = requests.post(f"{api_url}/generate", json=payload)
return response.json()
# Example usage
api_url = "http://localhost:8000"
result = query_vllm(api_url, "gpt-j", "Explain the concept of throughput in AI.")
print(result)
3. Parallelizing Requests
from concurrent.futures import ThreadPoolExecutor
def parallel_requests(func, args_list):
"""
Execute multiple requests in parallel using a thread pool.
"""
with ThreadPoolExecutor() as executor:
results = list(executor.map(func, args_list))
return results
# Define input prompts
prompts = ["Define AI.", "Explain NLP.", "What is LLM?"]
# Execute parallel queries for vLLM
responses = parallel_requests(
lambda prompt: query_vllm(api_url, "gpt-j", prompt),
prompts
)
print(responses)
Mathematical Calculations
Throughput and Latency

Python Code for Calculations
def calculate_metrics(total_time, num_requests):
"""
Calculate throughput and latency.
"""
throughput = num_requests / total_time
latency = (total_time / num_requests) * 1000
return throughput, latency
# Example
total_time = 10.0 # seconds
num_requests = 100
throughput, latency = calculate_metrics(total_time, num_requests)
print(f"Throughput: {throughput} TPS, Latency: {latency} ms")
Performance Benchmarks
1. Measuring Latency
import time
def measure_latency(func, *args):
"""
Measure latency for a single function call.
"""
start_time = time.time()
func(*args)
end_time = time.time()
return (end_time - start_time) * 1000 # in milliseconds
2. Visualizing Results
import matplotlib.pyplot as plt
# Sample benchmark data
tools = ["vLLM", "Ollama"]
latencies = [30, 50] # in ms
# Plot
plt.bar(tools, latencies, color=["blue", "green"])
plt.title("Latency Comparison")
plt.xlabel("Tool")
plt.ylabel("Latency (ms)")
plt.show()
Advanced Scenarios
Fine-Tuning with Ollama
def fine_tune_ollama(model_name, dataset_path):
"""
Fine-tune an Ollama model using a dataset.
"""
subprocess.run(["ollama", "fine-tune", model_name, dataset_path])
print("Fine-tuning complete.")
Scaling vLLM Requests
def scale_vllm_requests(api_url, model_name, prompt, num_requests):
"""
Scale vLLM requests by sending multiple prompts.
"""
responses = [
query_vllm(api_url, model_name, prompt) for _ in range(num_requests)
]
return responses
Conclusion
Both vLLM and Ollama cater to different audiences and use cases:
- Choose vLLM for production-grade applications where high throughput, low latency, and scalability are essential.
- Choose Ollama for offline prototyping, local inference, or scenarios where simplicity and privacy are critical.
The right tool depends on your project’s scale and requirements, but together, they showcase the power of diverse solutions for handling LLMs. What’s your preferred tool for LLM workflows? Let us know in the comments!