What role do ARG and ENV play in Dockerfile design? ARG defines variables at build time, which customizes the build process, while ENV establishes runtime configurations for containers. The choice between these options affects how Docker workflows meet specific requirements. This article examines the unique features of ARG and ENV with clear examples and practical advice for seamless integration into your development process.
What are ARG and ENV variables?
Dockerfiles offer developers powerful tools to manage container configurations during both build and runtime. ARG and ENV variables serve distinct but complementary purposes in these workflows, streamline the containerization process, and provide application flexibility. ARG and ENV also play important roles in optimizing cloud app development workflows. Dockerfiles benefit from the dynamic capabilities of ARG during image creation and the persistent nature of ENV to maintain runtime consistency.
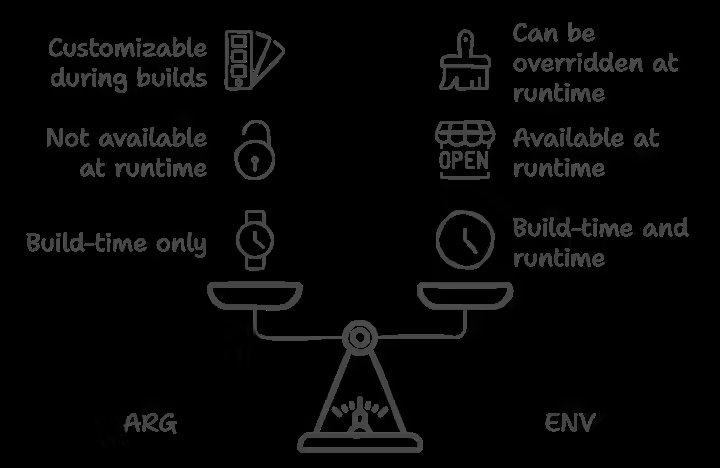
ARG build-time variables
The ARG instruction defines build-time variables that are only available during the image creation process. These variables can be declared with or without default values and are typically used to pass custom parameters during the build phase. For example, developers may use ARG to specify package versions, usernames, or other configuration parameters that need to vary based on the environment in which the image is built.
ARG APP_VERSION=1.0.0
RUN echo "Building version $APP_VERSION"
Here, the APP_ENV variable is available to the container when it starts, so that the application runs in the appropriate environment.
ENV persistent environment variables
The ENV instruction sets variables that persist throughout the lifecycle of the image and its containers. ENV variables are available both during the build phase and at runtime. This availability allows containers to access them during execution. These variables are suitable for configuration settings, paths, or other parameters that applications within the container require to function properly.
ENV APP_ENV=production
CMD echo "Running in $APP_ENV mode"
Here, the APP_ENV variable is available to the container when it starts, so that the application runs in the appropriate environment. Unlike ARG, ENV variables can also be overridden at runtime with the -e flag in the docker run command or via a .env file in docker-compose configurations.
- ARG variables are ephemeral and restricted to the build context. They enable dynamic customization of the image creation process but are inaccessible to running containers.
- ENV variables are permanent within the image and container lifecycle, which makes configuration consistent across environments.
Data from a 2022 Docker Usage Trends report shows that while 85% of developers use ENV for runtime configurations, ARG remains important for 65% of workflows requiring build-time customization
Feature |
ARG |
ENV |
---|---|---|
Scope |
Limited to the build stage; not accessible at runtime. |
Accessible throughout the container’s lifecycle. |
Modifiability |
Can be modified at build time using the –build-arg flag. |
Can be overridden at runtime using the -e flag or .env files. |
Persistence |
Temporary; discarded after the build process. |
Permanent; embedded into the image and container lifecycle. |
Security |
Visible in intermediate image layers; not suitable for sensitive data. |
Less secure if exposed but can store runtime configurations securely in .env files. |
Best Use Cases |
– Specifying software versions during build. – Dynamic base image selection. – CI/CD pipeline builds. |
– Application configurations like environment modes. – Setting paths and system-level variables. – Runtime customization for staging and production. |
Pros |
– Enables dynamic build-time customization. – Simplifies CI/CD workflows. |
– Persistent and accessible across container lifecycle. – Easily modifiable for runtime flexibility. |
Cons |
– Ephemeral nature limits runtime usability. – Unsuitable for sensitive data. |
– Embedded values may lead to larger images. – Requires careful handling to avoid exposing sensitive information. |
How to use Docker ARG in Dockerfile
Docker ARG is a versatile tool that shines in scenarios requiring dynamic inputs during the image build process. It allows developers to streamline workflows by injecting build-time variables without modifying the Dockerfile for each build. Here are the best use cases where ARG is indispensable.
1. Dynamic configuration for builds
One of the most common uses of ARG is injecting dynamic values into Dockerfiles to accommodate diverse project needs. This capability minimizes the need for redundant Dockerfiles and reduces maintenance complexity. When you deal with projects that rely on varying dependency versions, ARG simplifies switching between them:
ARG NODE_VERSION=16
FROM node:$NODE_VERSION
RUN echo "Building with Node.js $NODE_VERSION"
–build-arg flag allows developers easily specify the required version without editing the file:
docker build --build-arg NODE_VERSION=18 -t node_app
This approach is particularly useful in CI/CD pipelines where images for testing, staging, and production often require distinct dependencies.
2. Flexibility in multi-stage builds
Multi-stage builds often require variations in the base image to cater to different build environments. ARG offers the ability to dynamically switch between base images without hardcoding them into the Dockerfile.
ARG BASE_IMAGE=debian
FROM $BASE_IMAGE:latest AS builder
RUN apt-get update && apt-get install -y build-essential
This setup allows you to test builds across different Linux distributions, such as debian or alpine, by altering the BASE_IMAGE argument:
docker build --build-arg BASE_IMAGE=alpine -t app-alpine .
Such flexibility is invaluable for development teams managing multi-platform deployments or optimizing builds for specific environments.
3. CI/CD pipeline builds
In CI/CD workflows, tools such as Jenkins, GitHub Actions, or custom pipelines built with languages like Kotlin play important roles in automating builds. Docker ARG allows dynamic injection of branch names, commit hashes, or other context-specific data into the build process, enabling automation frameworks to tailor Docker images for specific branches or environments. A recent report by Red Hat highlights that 75% of enterprises that employ CI/CD pipelines use Docker ARG to optimize builds for specific branches and deployment stages.
docker build --build-arg BRANCH_NAME=feature-auth -t app-feature-auth .
The BRANCH_NAME variable customizes the build process to tag or configure the image based on the current development branch. Automation tools like Jenkins or GitHub Actions can pass these arguments programmatically during the build stage:
steps:
- name: Build Docker Image
run: docker build --build-arg BRANCH_NAME=${{ github.ref_name }} -t myapp:${{ github.sha }} .
This streamlines development workflows by automating variable injection, improves traceability with tags tied to branch names or commits and reduces manual intervention and the risk of errors in dynamic builds.
How to Use Docker ENV in Dockerfile
Docker ENV serves as a foundational tool to define environment variables in Dockerfiles. These variables persist throughout the container’s lifecycle, which makes ENV best to configure applications and maintain consistent runtime behavior. Here are its key uses.
1. Set persistent environment variables
ENV variables are often used to define application-specific configurations that must remain accessible during the container’s runtime.
ENV APP_ENV=development
ENV DATABASE_URL=postgres://db_user:db_pass@localhost:5432/mydb
The APP_ENV variable sets the environment mode (e.g., development, production). DATABASE_URL specifies the database connection string. It provides a single source of truth for runtime configurations and simplifies debugging by allowing easy modification of environment-specific settings.
2. Define paths and system-level variables
Another use of ENV is to define paths for system binaries, libraries, or configuration files. This makes the container operate with the correct dependencies and file structures, maintaining consistency across deployments. Consider the following example:
ENV PATH="/custom/bin:$PATH"
ENV CONFIG_DIR="/app/config"
Here, the PATH variable is modified to include a custom directory, allowing the container to locate and execute custom binaries. Similarly, CONFIG_DIR specifies the default location for application configuration files. This approach standardizes file locations and provides compatibility with custom setups or additional tools required by the application.
3. Configure applications for multiple environments
Applications often require distinct configurations for development, staging, and production environments. Docker ENV allows developers to set default values that adapt to different deployment needs, providing a seamless transition between these environments. For instance:
ENV NODE_ENV=production
ENV LOG_LEVEL=info
Here, NODE_ENV is set to production for optimized performance, while LOG_LEVEL specifies the verbosity of application logs. This flexibility enables teams to maintain consistent application behavior across environments while allowing runtime modifications for testing or diagnostics.
Can you use ARG and ENV together
Docker ARG and ENV can be combined to create a seamless workflow between build-time and runtime configurations. While ARG variables are temporary and only available during the image build process, ENV variables persist into the runtime environment. This combination allows developers to pass values dynamically from build-time to runtime, which improves both flexibility and maintainability.
One common approach is initializing ENV variables with ARG values during the build process. This allows developers to define dynamic defaults at build-time while maintaining their persistence at runtime. For instance, a build-time ARG can specify the default port for an application, and an ENV variable can retain this value for runtime usage. This setup eliminates the need to duplicate configurations, which makesDockerfiles cleaner and more maintainable. Here’s an example:
ARG APP_PORT=3000
ENV PORT=$APP_PORT
In this example, the ARG variable APP_PORT provides a default value, which is then passed to the ENV variable PORT.
Another practical application is to use ARG to inject sensitive or environment-specific values during the build stage without permanently embedding them into the image. For example, developers can pass build-time arguments for repository URLs or API keys and transfer them to ENV variables when runtime processes require them. However, do this cautiously, as ARG values can remain visible in intermediate image layers. To mitigate risks, ARG is generally recommended for temporary, non-sensitive data.
In multi-stage builds, combining ARG and ENV streamlines complex workflows. Developers can define ARG variables to toggle between different stages and environments and initialize ENV values based on these inputs. For example, an ARG variable can determine the base image in the first stage, and the same value can influence runtime behavior through ENV in subsequent stages. This approach optimizes both build-time flexibility and runtime stability, which is especially valuable in CI/CD pipelines where reproducibility and adaptability are important.
Conclusion
Developers who understand the unique properties of ARG and ENV can streamline their Docker workflows, which will improve both security and efficiency. Choose between ARG and ENV based on your application’s specific requirements. Use ARG for build-time flexibility and security, and ENV for runtime configuration. A combination of both creates Dockerfiles that meet your app development needs.