Docker Terraform Provider is a new tool designed to streamline the way users and organizations manage Docker-hosted resources. This includes managing repositories, configuring teams, and defining organization settings, all within Terraform’s infrastructure-as-code ecosystem. With this provider, Docker resources are now easier than ever to automate, secure, and scale, whether you’re managing a small project or a complex organizational setup.
A New Era of Working with Docker Hub

The Docker Terraform Provider presents a transformative way to interact with Docker Hub, bringing in the best practices of infrastructure-as-code that are foundational to cloud-native environments. Integrating Docker Hub with Terraform enables organizations to manage resources efficiently, improve security protocols, and collaborate more effectively across teams, all while synchronizing Docker components with other infrastructure.
The Challenge
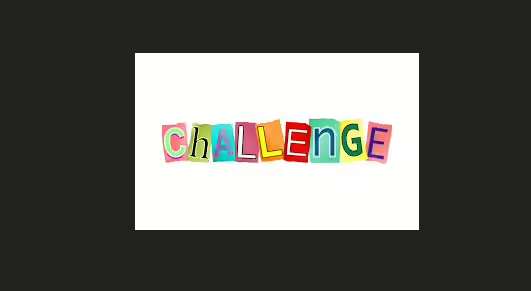
For many teams, managing Docker Hub resources manually can be time-consuming and error-prone, especially as projects expand. Configurations managed manually often lead to inconsistencies, weakened security, and limited collaboration without version control. This is where the Docker Terraform Provider makes a difference, allowing Docker Hub resources to be managed as code, thus enhancing consistency, auditability, and automation.
The Solution
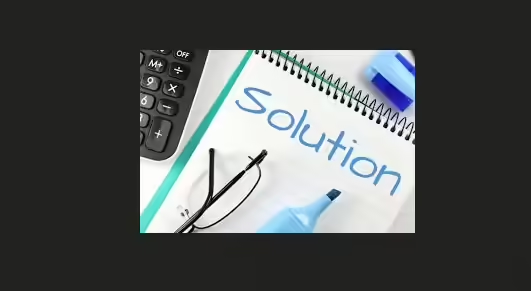
The Docker Terraform Provider introduces the following features:
- Unified Management: Use the same code and structure to manage Docker repositories, teams, users, and organizations across environments.
- Version Control: Changes to Docker Hub resources are tracked within your Terraform configuration, providing an auditable, version-controlled system for Docker infrastructure.
- Collaboration and Automation: Teams can seamlessly automate the provisioning and management of Docker Hub resources using Terraform, enhancing both productivity and adherence to best practices.
- Scalability: Whether managing a single repository or an entire organization, the Docker Terraform Provider effortlessly scales to meet your needs.
Example Use Case
The Docker Terraform Provider now empowers anyone in the company to create repositories directly from code without requiring elevated permissions on Docker Hub. By reducing these permissions and streamlining workflows, the new provider has boosted developer efficiency and strengthened security protocols.
Here’s an example Terraform configuration managing a repository, a team, team permissions, and a Personal Access Token (PAT):
terraform {
required_providers {
docker = {
source = "docker/docker"
version = "~> 0.2"
}
}
}
# Initialize provider
provider "docker" {}
# Define local variables for customization
locals {
namespace = "my-docker-namespace"
repo_name = "my-docker-repo"
org_name = "my-docker-org"
team_name = "my-team"
my_team_users = ["user1", "user2"]
token_label = "my-pat-token"
token_scopes = ["repo:read", "repo:write"]
permission = "admin"
}
# Create repository
resource "docker_hub_repository" "org_hub_repo" {
namespace = local.namespace
name = local.repo_name
description = "This is a generic Docker repository."
full_description = "Full description for the repository."
}
# Create team
resource "docker_org_team" "team" {
org_name = local.org_name
team_name = local.team_name
team_description = "Team description goes here."
}
# Team association
resource "docker_org_team_member" "team_membership" {
for_each = toset(local.my_team_users)
org_name = local.org_name
team_name = docker_org_team.team.team_name
user_name = each.value
}
# Create repository team permission
resource "docker_hub_repository_team_permission" "repo_permission" {
repo_id = docker_hub_repository.org_hub_repo.id
team_id = docker_org_team.team.id
permission = local.permission
}
# Create access token
resource "docker_access_token" "access_token" {
token_label = local.token_label
scopes = local.token_scopes
}
Real Life Use case Implementation
First we would consider using Linux for our implementations, after which we would proceed to configuring docker password setup in order for the password to be stored in the computer since terraform will have to communicate with the stored password, Docker may rely on the pass
tool for secure credential storage. You can initialize it , or alternatively, use a different method for Docker credentials if you don’t want to set up pass
.
Initialize pass
- Install
pass
if it’s not already installed:sudo apt update sudo apt install pass
- Initialize
pass
with a GPG key. If you don’t already have a GPG key, you’ll need to create one. You can do so with this command:gpg --full-generate-key
Follow the prompts to set up your GPG key.
- Once you have a GPG key, initialize
pass
by providing your GPG key ID. You can list available keys with:gpg --list-keys
Then initialize
pass
:pass init "YOUR_GPG_KEY_ID"
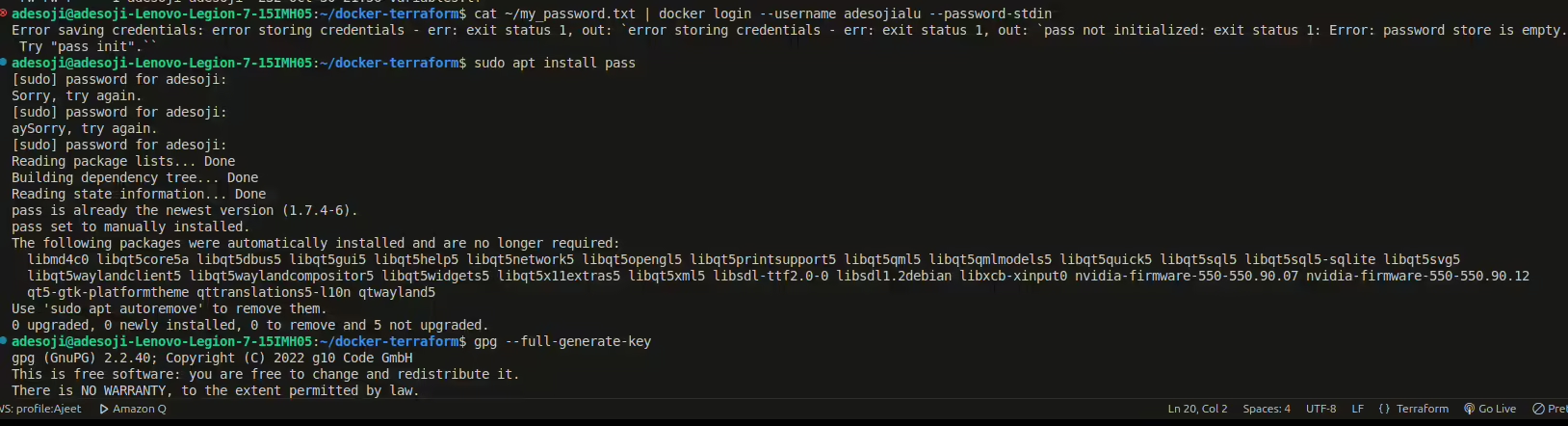
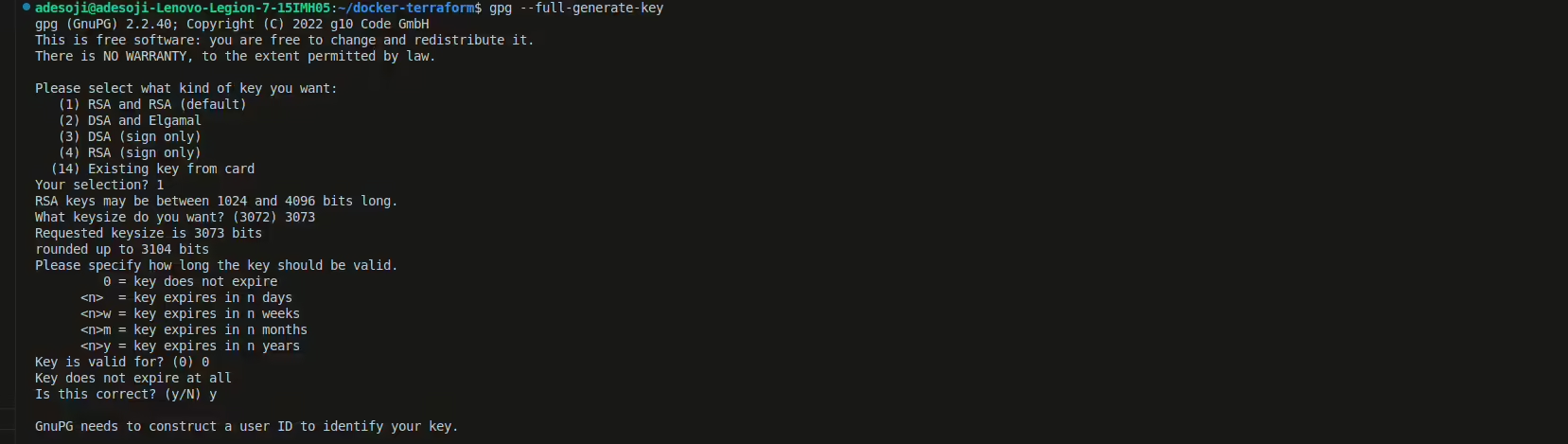
Now, having completed the above, proceed below to install docker desktop (DD) for various OS architecture, then we verify docker version
- Prerequisites
- Install DD (Windows, macOS, Linux)
- Set Up the Docker Terraform Provider
- Configure Terraform Resources Line-by-Line
Prerequisites
Before you start, make sure you have:
- Docker Hub Account: Sign up at Docker Hub.
- DD: Installed and running on your machine.
- Terraform: Installed on your machine. Follow instructions from the Terraform download page.
For macOS & Linux: Use Homebrew or a package manager for Terraform.
# macOS/Linux using Homebrew
brew install terraform
For Windows: Download the binary from the Terraform website and add it to your PATH.
Install DD
DD enables you to run Docker in a local development environment. Choose your operating system for the installation steps:
DD for Windows
- Download DD from Docker’s official website.
- Double-click the installer and follow the instructions.
- Once installed, launch DD and ensure it is running.
DD for macOS
- Download DD for Mac from Docker’s download page.
- Drag the Docker app to your Applications folder.
- Launch DD and verify it’s running in the menu bar.
DD for Linux
- Follow the installation instructions specific to your Linux distribution from Docker’s documentation page.
- Start DD from your applications menu or by running:
systemctl start docker
After DD is running, verify Docker functionality by running:
docker --version

Having verified our docker version from the image above, we proceed to login into docker
Docker Login
Save your Docker password in a secure file (optional but recommended for security):

echo "my-secret-password" > ~/my_password.txt
Once pass
is initialized, run the docker login
command again:
cat ~/my_password.txt | docker login --username xxxxxxx --password-stdin
This should now save the Docker credentials in the pass
store, and the docker
CLI will have access to them as you can see login succeeded in the image above.
Alternatively: Use Environment Variables Directly
If you prefer not to use pass
, you can skip docker login
and instead set environment variables for Terraform, Replace xxxxxx below with your own username and passowrd for docker:
export DOCKER_USERNAME="xxxxxx"
export DOCKER_PASSWORD="xxxxxxx"
This approach lets Terraform use the specified environment variables without saving them in Docker’s credential store,But in this blog, we used the docker credentials.
Set Up the Docker Terraform Provider
Here, we’ll create a Terraform configuration to define Docker Hub resources. Start with initializing your Terraform environment:
- Create a Project Directory:
mkdir docker-terraform && cd docker-terraform
- Initialize Terraform:
Inside your project directory, create a new file called
main.tf
, and add the provider configuration:terraform { required_providers { docker = { source = "docker/docker" version = "~> 0.2" } } } provider "docker" {}
This tells Terraform to use the Docker provider, pulling it from the Terraform registry.
- Initialize the Provider:
Run the following command to download the provider plugins:
terraform init
Configuring Docker Resources: Line-by-Line Guide
Now, let’s define Docker resources with explanations for each line. In main.tf, add resource definitions based on your Docker setup.
Set Local Variables
locals {
namespace = "my-docker-namespace"
repo_name = "my-docker-repo"
org_name = "my-docker-org"
team_name = "my-team"
my_team_users = ["user1", "user2"]
token_label = "my-pat-token"
token_scopes = ["repo:read", "repo:write"]
permission = "admin"
}
– namespace
: Docker namespace or username.
– repo_name
: Name of the repository to be created.
– org_name
: Organization name in Docker Hub.
– team_name
: The name of the team within Docker Hub.
– token_label
& token_scopes
: Used for generating access tokens with specified permissions.
Create a Docker Repository
resource "docker_hub_repository" "org_hub_repo" {
namespace = local.namespace
name = local.repo_name
description = "This is a generic Docker repository."
full_description = "Full description for the repository."
}
– This block creates a Docker repository with specified attributes like namespace
, name
, and descriptions.
Define a Team
resource "docker_org_team" "team" {
org_name = local.org_name
team_name = local.team_name
team_description = "Team description goes here."
}
– This defines a Docker organization team, which enables role-based access within Docker Hub.
Assign Users to the Team
resource "docker_org_team_member" "team_membership" {
for_each = toset(local.my_team_users)
org_name = local.org_name
team_name = docker_org_team.team.team_name
user_name = each.value
}
– The loop iterates over users defined in my_team_users
, associating each one with the team.
Set Permissions for the Team on the Repository
resource "docker_hub_repository_team_permission" "repo_permission" {
repo_id = docker_hub_repository.org_hub_repo.id
team_id = docker_org_team.team.id
permission = local.permission
}
– This block assigns the specified permission (e.g., admin
) to the team on the repository.
Create a Personal Access Token (PAT)
resource "docker_access_token" "access_token" {
token_label = local.token_label
scopes = local.token_scopes
}
– This generates a PAT, essential for accessing the repository programmatically with defined scopes (repo:read
, repo:write
). having created all these sub terraform configurations, we proceed to applying the Plan for the Infrastructure setup
Run the Terraform Plan
To view the Plan your configuration,execute:
terraform Plan

Having accepted the plan , the next step is to run the terraform apply as seen in the image below :

Apply the Terraform Configuration
To apply your configuration and provision these resources on Docker Hub, execute:
terraform apply
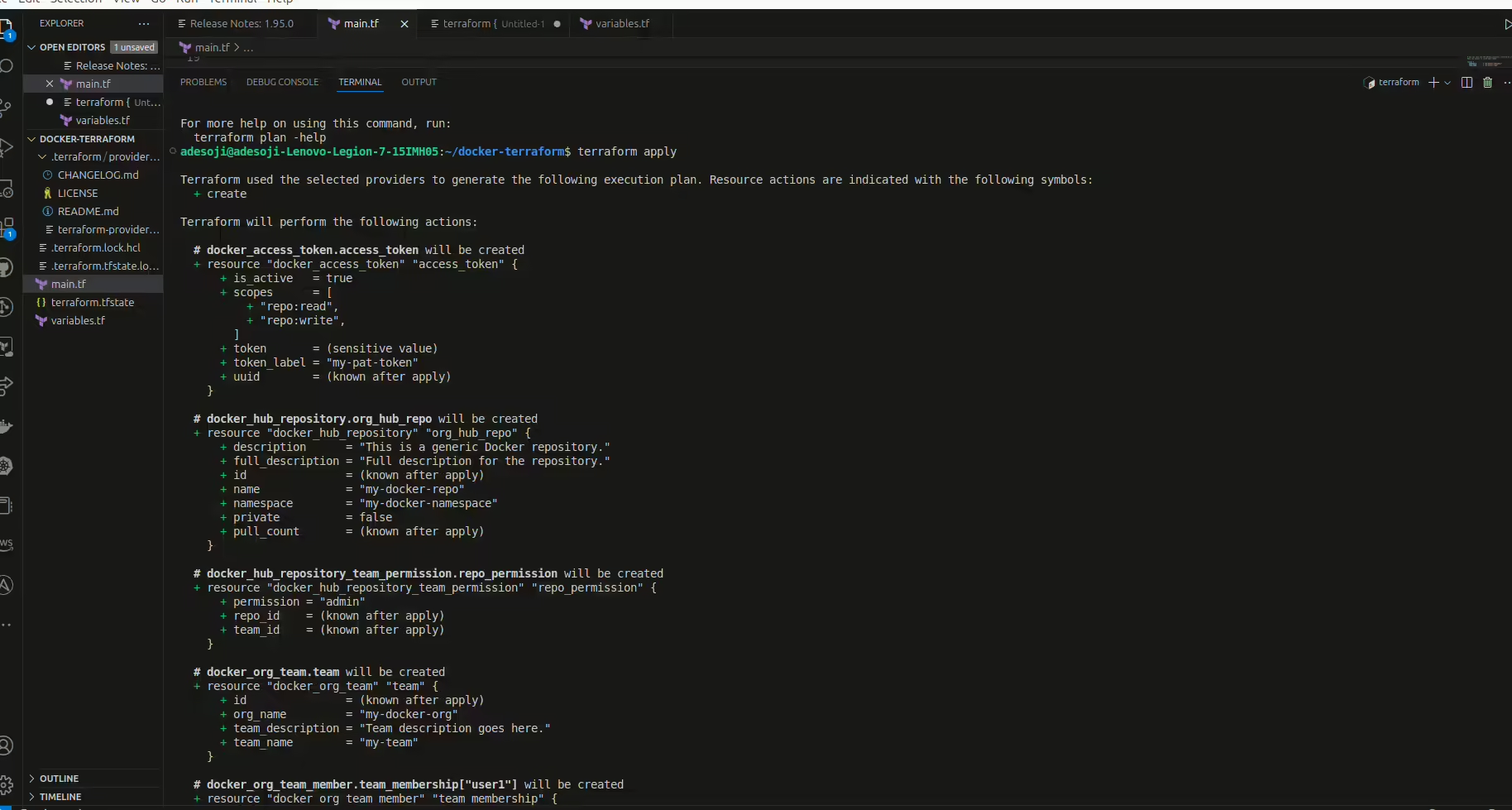
Review the proposed changes, then confirm by typing yes
as seen in the image below.

The full source code can be found in this GitHub repository: here
This guide provides a foundational setup for using the Docker Terraform Provider to manage Docker resources on Docker Hub. For more advanced configurations, consider exploring module structures and CI/CD automation to streamline Terraform management across multiple environments. Each configuration step outlined here will help you understand and customize these setups for future Docker Terraform projects.
Our work with the Docker Terraform Provider is just getting started. We’re planning to integrate additional Docker services like Docker Scout, Docker Build Cloud, and Testcontainers Cloud. These enhancements will provide greater flexibility and control, ensuring that as your Docker setup grows, the Terraform provider can scale to meet new demands.Connect with Collabnix to stay updated.
Stay Updated
To keep up with the latest improvements and community discussions, check out the official Docker Terraform Provider repository. Track issues, share feedback, and follow new feature requests directly through the issue tracker.